Background Geolocation Tracking with Ionic 5+ PouchDB
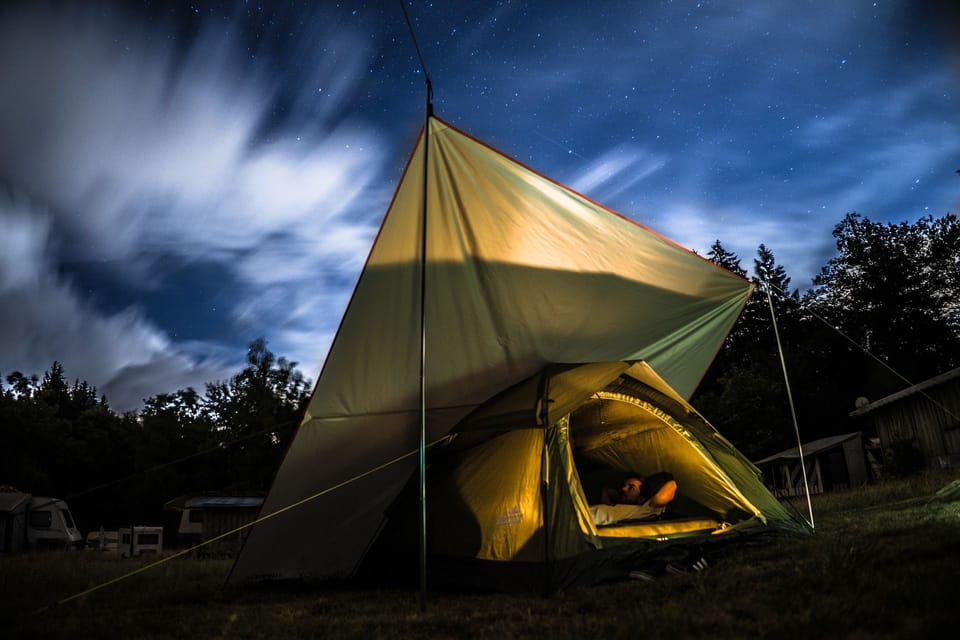
Step 1
Create a new Ionic project.
npm install -g ionic@latest
ionic start ionic4-background-geo-pouchdb blank
Step 2
Install plugin and dependencies.
// geolocation tracking
ionic cordova plugin add @mauron85/cordova-plugin-background-geolocation
npm install --save @ionic-native/background-geolocation
// sqlite as storage without limits
ionic cordova plugin add cordova-sqlite-storage
npm install @ionic-native/sqlite
// pouchDB
npm install pouchdb-adapter-cordova-sqlite --save
npm install pouchdb --save
That's all what you need to install in order to start using PouchDB with SQLite in your Ionic app without any storage limits.
Step 3
Create Couch Provider
import { Injectable } from '@angular/core';
import * as PouchDB from 'pouchdb/dist/pouchdb';
import * as cordovaSqlitePlugin from 'pouchdb-adapter-cordova-sqlite/dist/pouchdb.cordova-sqlite';
@Injectable({
providedIn: 'root'
})
export class CouchApiService {
constructor() {
PouchDB.plugin(cordovaSqlitePlugin);
}
addEntry(name, entry) {
const db = new PouchDB(name + '.db',
{
auto_compaction: true,
adapter: 'cordova-sqlite',
iosDatabaseLocation: 'Library',
androidDatabaseImplementation: 2,
timeout: 15000,
batch_size: 500
});
return db.post(entry);
}
}
Step 4
Add to app.module.ts
import { BackgroundGeolocation } from "@ionic-native/background-geolocation/ngx";
import { CouchApiService } from './providers/couch-api.service';
providers: [
…
BackgroundGeolocation,
CouchApiService,
],
home.page.ts
import {
BackgroundGeolocation,
BackgroundGeolocationConfig,
BackgroundGeolocationResponse,
BackgroundGeolocationEvents
} from "@ionic-native/background-geolocation/ngx";
import * as PouchDB from 'pouchdb/dist/pouchdb';
import * as cordovaSqlitePlugin from 'pouchdb-adapter-cordova-sqlite/dist/pouchdb.cordova-sqlite';
import { CouchApiService } from '../providers/couch-api.service';
@Component({
selector: 'app-home',
templateUrl: 'home.page.html',
styleUrls: ['home.page.scss']
})
export class HomePage{
constructor(
private readonly couchApiService: CouchApiService,
private readonly backgroundGeolocation: BackgroundGeolocation
) {
PouchDB.plugin(cordovaSqlitePlugin);
this.startBackgroundGeolocation();
}
startBackgroundGeolocation() {
const config: BackgroundGeolocationConfig = {
desiredAccuracy: 10,
stationaryRadius: 20,
distanceFilter: 100,
debug: true, // enable this hear sounds for background-geolocation life-cycle.
stopOnTerminate: false // enable this to clear background location settings when the app terminates
};
this.backgroundGeolocation.configure(config).then(() => {
this.backgroundGeolocation
.on(BackgroundGeolocationEvents.location)
.subscribe((location: any) => {
if (location.speed == undefined) {
location.speed = 0;
}
// add location into pouchDB
this.couchApiService.addEntry('MyDatabaseName', location)
.then(data => {
this.backgroundGeolocation.finish(); // FOR IOS ONLY
})
.catch(error => {
this.backgroundGeolocation.finish(); // FOR IOS ONLY
});
// IMPORTANT: You must execute the finish method here to inform the native plugin that you're finished,
// and the background-task may be completed. You must do this regardless if your operations are successful or not.
// IF YOU DON'T, ios will CRASH YOUR APP for spending too much time in the background.
});
});
// start recording location
this.backgroundGeolocation.start();
}
Step 5
Add platform
ionic cordova platform add android
ionic cordova prepare android
ionic cordova platform add ios
ionic cordova prepare ios
The End
If you found any problem following the tutorial, please leave your comment below.
If this post was helpful, please click the ❤️ and ? button below a few times to show your support! ⬇⬇