How to stream YouTube videos in Ionic
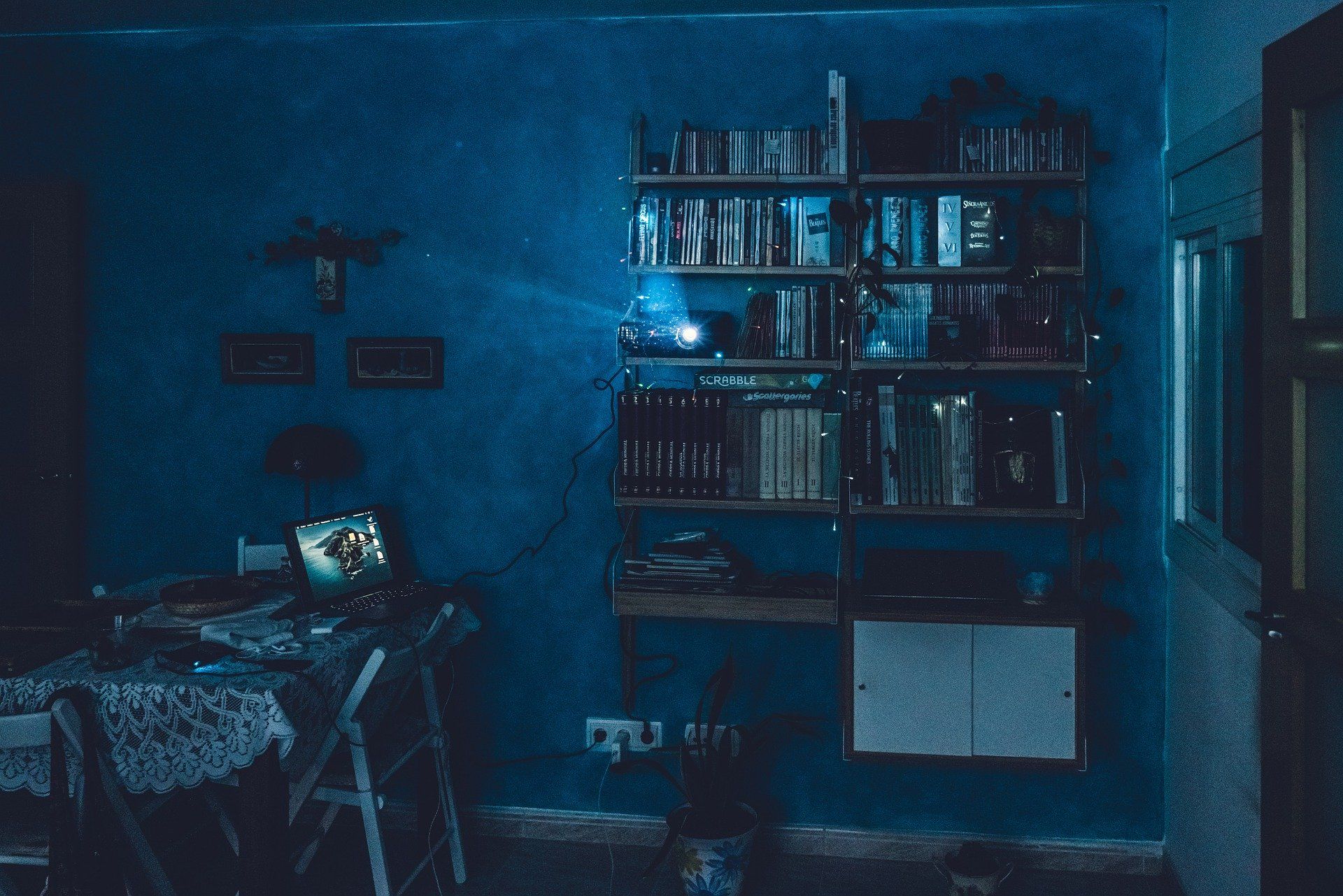
How it works
Step 1
Create an ionic angular app or use an existing one. We will create a new one using the "tabs" template.
ionic start myApp tabs
Step 2
Install the following dependencies
ionic cordova plugin add cordova-plugin-advanced-http
ionic cordova plugin add cordova-plugin-streaming-media
npm install ionic-youtube-streams --save
npm install @ionic-native/streaming-media --save
https://www.npmjs.com/package/ionic-youtube-streams
Step 3
Open the app in your IDE
Step 4
Import the "ionic-youtube-streams" dependency in your *.page.ts
import * as yt from 'ionic-youtube-streams';
import {StreamingMedia, StreamingVideoOptions} from '@ionic-native/streaming-media/ngx';
Step 5
Create a method for fetching the youtube stream based on a video id. Then create a second method for playing the stream.
constructor(private readonly streamingMedia: StreamingMedia) {}
async streamVideo() {
const info: any = await yt.info('XJNlK8n_rTU');
this.streamURL(info.formats[0].url);
}
private streamURL(url: any) {
const options: StreamingVideoOptions = {
successCallback: () => {
},
errorCallback: (e) => {
console.log('Error streaming');
},
orientation: 'portrait',
shouldAutoClose: true,
controls: true
};
this.streamingMedia.playVideo(url, options);
}
Step 6
Add the StreamingMedia Provider to your app.module.ts
import {StreamingMedia} from "@ionic-native/streaming-media/ngx";
providers: [
...
StreamingMedia,
],
Step 7
Create a UI element e.g. a button, which will start playing the video stream
<ion-button expand="block" (click)="streamVideo()">Stream Video</ion-button>
Step 8
Start the app in your emulator and test
ionic cordova emulate ios -l -c --target "YOUR SIMULATOR ID" -- --buildFlag="-UseModernBuildSystem=0"