How to track Ionic Apps with Google Analytics - no cordova plugin
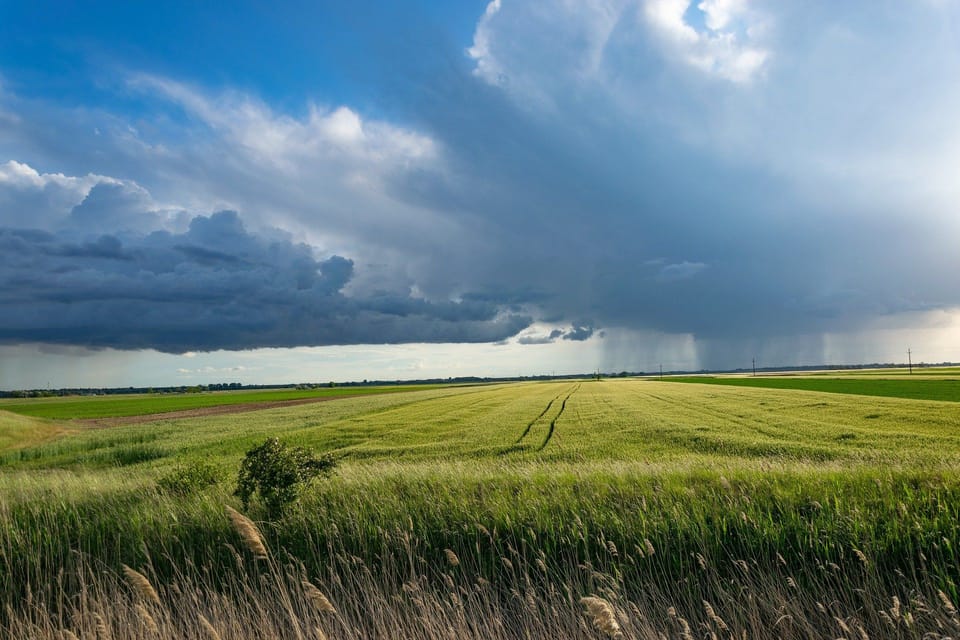
In Short:
You will learn how to add Google Analytics without cordova plugin and track page transitions and your own events
Pre-Condition:
You have created a Google Analytics account and also have registered a website based project for your ionic app. Also you have extracted your Tracker Id from your GA code tracking snippet.
The Tracker format has the following syntax:
'XX-XXXXXXXX-X'
How it works
Step 1
Install the following dependencies
npm install --save @types/google.analytics
Step 2
Add the following snippet to your index.html
<script>
(function(i,s,o,g,r,a,m){i['GoogleAnalyticsObject']=r;i[r]=i[r]||function(){
(i[r].q=i[r].q||[]).push(arguments)},i[r].l=1*new Date();a=s.createElement(o),
m=s.getElementsByTagName(o)[0];a.async=1;a.src=g;m.parentNode.insertBefore(a,m)
})(window,document,'script','https://www.google-analytics.com/analytics.js','ga');
</script>
Step 3
Create a new Provider for handling the Google Analytics interactions and import it to your app.module.ts file
import { AnalyticsService } from './analytics.service';
...
@NgModule({
...
providers: [
...
AnalyticsService,
...
]
})
import { Injectable } from '@angular/core';
declare var ga;
@Injectable({
providedIn: 'root'
})
export class AnalyticsService {
constructor() { }
setTracker(tracker) {
if ( !localStorage.getItem('ga:clientId') ) {
localStorage.setItem( 'ga:clientId', tracker.get('clientId') );
}
}
startTrackerWithId(id) {
ga('create', {
storage: 'none',
trackingId: id,
clientId: localStorage.getItem('ga:clientId')
});
ga('set', 'checkProtocolTask', null);
ga('set', 'transportUrl', 'https://www.google-analytics.com/collect');
ga(this.setTracker);
}
trackView(pageUrl: string, screenName: string) {
ga('set', {
page: pageUrl,
title: screenName
});
ga('send', 'pageview');
}
trackEvent(category, action, label?, value?) {
ga('send', 'event', {
eventCategory: category,
eventLabel: label,
eventAction: action,
eventValue: value
});
}
}
Step 4
Init your app with your Google Analytics tracker id in the app.component.ts
Also you can register on the Angular router events and track switches between pages automatically
...
import { AnalyticsService } from './analytics.service';
import { Router, NavigationStart } from '@angular/router';
import { Title } from '@angular/platform-browser';
@Component({
selector: 'app-root',
templateUrl: 'app.component.html'
})
export class AppComponent {
constructor(
...
private analyticsService: AnalyticsService,
public router: Router,
private title: Title,
) {
this.initializeApp();
}
initializeApp() {
//Start track passing Tracker Id
this.analyticsService.startTrackerWithId('XX-XXXXXXXX-X');
...
this.router.events
.subscribe(event => {
//observe router and when it start navigation it will track the view
if (event instanceof NavigationStart) {
let title = this.title.getTitle();
//get title if it was sent on state
if (this.router.getCurrentNavigation().extras.state) {
title = this.router.getCurrentNavigation().extras.state.title;
}
//pass url and page title
this.analyticsService.trackView(event.url, title);
}
});
}
}
Step 5
For creating specific events. Add the following code to your services:
import { AnalyticsService } from './analytics.service';
export class MyServicePage {
constructor(
public analytics: AnalyticsService,
) {}
playSoundExample() {
this.analytics.trackEvent('User Interaction', 'Play Sound', 'Super Mario Sound');
}
}
Step 6 (Optional)
Also you can add specific title descriptions for page transitions
<a routerLink="/items/123" [state]="{ title: 'Test Link' }" routerDirection="root">
My test link
</a>